Once you’ve handed over a WordPress website to a client, it should be champagne for everyone.
Unfortunately, in reality, Murphy’s Laws tends to take precedence over champagne moments. There are plenty of things that can (and do) go wrong after handover, and the client may very well be the person to unintentionally nuke his own site. All it takes is a bit of innocent tinkering with the theme files, plugins, or maybe even widgets, and suddenly you will have a problem where there shouldn’t be one.
As WordPress adds more power and the potential of complexity develops in the dashboard, it is important to consider an approach similar to encapsulation in object-oriented programming by making the power of WordPress available to clients while selectively hiding key functions in order to protect the site from accidental corruption.
In this post I want to give you a number of tips and best practices that will enable you to achieve something close to that. So without further ado, let’s crack on with creating a WordPress back end that is safe for (and from!) clients.
Get Started with Best Practices
Before we go into the gritty details, it will serve us well to look at some best practices for security – even if they are seemingly unrelated to the topic at hand.
Basic Security Measures
A website that has the basics covered is more likely to be safe(r). So, make sure you’ve executed the simplest of security measures at a bare minimum, including:
- replacing the “admin” username,
- changing the login page from the default wp-login.php, and
- adding brute force login protection.
For more information, take a look at Nick’s WordPress security guide. And remember: A strong password is your first line of defence, so always pick one that’s as strong as a horse.
User Roles
The next thing on your list should be handling of user roles in WordPress.
If it can be avoided, keep the administrator role to yourself. In an ideal world, there should only be one administrator. In a multisite installation, keep both the Super Administrator and Administrator accounts stashed away.
The administrator shouldn’t be a regular work profile; instead, encourage the client to use another account with different privileges.
Users can be assigned various roles to fit their functions on the site. Here’s a quick look at various WordPress user roles and what they can perform.
- The SuperAdmin (available on multisite installations only) has full access to the site network.
- The Administrator has access to all features within a single site.
- An Editor can take control over the publishing and management of all posts and pages on a website, and additionally perform comments moderation without limitation.
- An Author can manage, publish and edit his own posts.
- A Contributor can manage his own posts, but cannot publish them.
- A Subscriber only has read privileges, and cannot make any changes to the website.
The default role of a new user can be applied in Settings > General from the WordPress dashboard. Administrators can view and change the roles of all users by navigating to All Users > Users.
Simplify the Dashboard for the Client
Simplifying the dashboard has multiple benefits. You get to declutter the screen to free up some real estate, and you get to hide potentially damaging options like plugin and theme editors.
The WordPress dashboard’s sidebar is full of items and options. Setting up roles for users will do away with several of these, but there may be a situation where you would prefer to remove some other items of your choice. This is where you use remove_menu_page() and remove_submenu_page(). You just need to add some code to your functions.php file:
// Remove specific menu items function remove_menus(){ if ( !current_user_can( 'manage_options' ) ) { remove_menu_page( 'tools.php' ); } } add_action( 'admin_menu', 'remove_menus' );
This code will remove the Tools menu for anyone who isn’t the administrator. You can use the current_user_can() function to selectively choose items for user roles. The key item to remember here is the menu slug. WordPress menu names are pretty descriptive for the slugs, but some have minor changes. This WordPress Codex example has a list of all menu slugs and the associated menus.
Keep in mind that these actions aren’t supposed to remove these options for the administrator, and thus apply only to users at the Editor level and below. Also, these options don’t really remove the menus; they hide them. Clients could still access those options through a direct hyperlink.
Add Help and Pointers
It can be useful to offer quick pointers and help to the client on the dashboard, and encourage them to call you – the developer – rather than attempt to make changes themselves (even if they seem trivial). Adding a widget is a great way to let the client know where to find the right information.
// Add a custom dashboard widget function textfor_dashboard_widget( $post, $callback_args ) { echo " Hello Mr. Client, remember to contact your developer before you make changes to the plugin or theme. <ul> <li>Website</li> <li>Phone Number</li> </ul> "; } function add_dashboard_widgets() { wp_add_dashboard_widget('dashboard_widget', 'Hello Mr. Client', 'textfor_dashboard_widget'); } add_action('wp_dashboard_setup', 'add_dashboard_widgets' );
This is how your widget will look:
Another useful thing would be adding help text to various menu items and functions, to let the client know exactly what to expect from the item they are about to use. Custom text can be a huge help, as you can focus on the requirements of the clients. The add_help_tab() function is what you need.
// Add help text to a specific page function adding_help_tab() { $screen = get_current_screen(); if ( 'post' == $screen->post_type ) { get_current_screen()->add_help_tab( array( 'id' => 'post', 'title' => ( 'Writing Guidelines' ), 'content' => '<strong>Hello, please keep these writing guidelines in mind.</strong> <ul> <li>The content you add must be unique.</li> <li>Add relevant and exciting images with your content.</li> <li>Remember to add title, alt text, and a descriptive caption for your images.</li> </ul> ', ) ); } } add_action( 'admin_head', 'adding_help_tab' );
And this is how it will look:
Disable Theme and Plugin Editing
We can hide the theme and plugin editing options, but that may not necessarily be enough; it may smarter to do disable editing altogether.
A quick way of preventing theme editing is to set the user permission on files to ‘644’ using either chmod or an FTP client. Changes to the theme will now require the use of an FTP client.
However, a more elegant way that covers themes as well as plugins is possible with the wp-config.php file. All you need to do is add one line:
define( 'DISALLOW_FILE_EDIT', true );
Redirect Non-Admin Users
This option is especially useful for sites that may otherwise have several people accessing the dashboard.
Simple authors and contributors could be simply whisked away to a custom page for submitting their content, rather than being allowed into the dashboard. This will require the following PHP code in your functions.php file:
// Redirect non-admin users away from dashboard to a custom url add_action( 'init', 'redirect_non_administrator' ); function redirect_non_administrator() { //Check if the user is the administrator if ( is_admin() && ! current_user_can( 'administrator' ) && //Make sure AJAX calls aren't blocked by the redirect ! ( defined( 'DOING_AJAX' ) && DOING_AJAX ) ) { //Replace my_custom_url with the target url wp_redirect( 'my_custom_url' ); exit; } }
Keeping dashboard access to only a specific few will automatically translate into a safer back end for the website.
Get Plugins on the Job
You could make quick, small changes with code, but if some sweeping changes are the order of the day, get plugins to do the job. They will allow you the flexibility to either remove a few items, or go the whole nine yards and white label the dashboard. Here are a couple of plugins to consider:
White Label CMS
White Label CMS is a very popular and free plugin to customize the dashboard. The plugin enables you to customize dashboard panels and logos and remove menus, and also grants Editor access to certain menus and widgets.
The plugin’s settings offers four major options:
Branding will enable you to make cosmetic changes like:
- swapping the WordPress logo with your own,
- adding information to the footer, and
- introducing a custom CSS for the login page.
True to its name, the Dashboard Panels screen enables you to control which panels to show and hide. You can also create a couple of custom widgets or add an RSS widget.
The Admin Settings can be used to allow a login URL redirect, or hide things like the Nag Update, Screen Options and Help Box. You can also clear up the clutter of meta boxes in page and post options, which should come as a relief. The option also allows custom CSS for the WordPress admin.
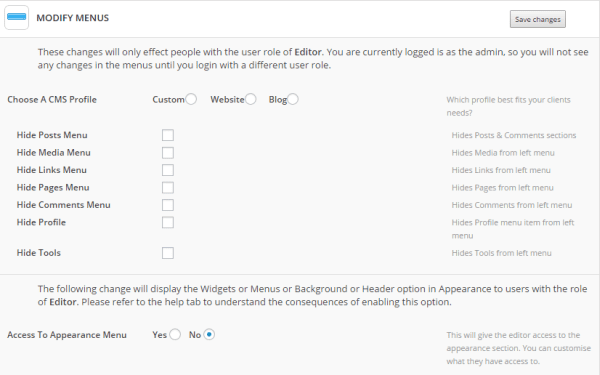
The Modify Menus Option of White Label CMS
The Modify Menus option is a major player here, and very relevant to our current discussion. It enables you to decide which menu options should be available to the Editor user role. This box offers two custom profiles – Website or Blog – to make the recommended menu items available to the user, or you can select custom items. There’s also an option to grant the Editor access to the Appearance menu, which you should steer clear of unless you understand the risks involved.
Ultimate Branding
It is only fair that we add a paid/premium plugin to match the free offering above. Ultimate Branding is a powerful plugin that enables you to modify and rebrand the dashboard and login page. Apart from cosmetic changes like branding and colors, it grants control over dashboard widgets, user role specific menu items, fonts, customized help content area, plus many more major and minor changes.
Ultimate Branding – as the name implies – is more about modifying the dashboard to create a custom appearance. That however, does not mean that this plugin skips over essential functionality. The settings area of the plugin offers five options: Dashboard, Text Change, Images, Admin Bar and Widgets:
It performs similar functions to White Label CMS but is more powerful and has more options on offer (as you would expect from a premium plugin). For example, you can even change the conventional WordPress greeting (“Howdy, username”) to your own preference, or make bigger changes like deciding what widgets to show and creating a custom appearance for the dashboard.
Price: $19 monthly | More Information
Complete Customization with Dashboard Themes
Dashboard Themes have been getting a lot of attention recently, and only seem to be growing in popularity.
They currently operate in a limbo state between actual themes and plugins. Most of these themes actually are plugins, but it’s the direction they are taking that sets them apart.
Here are a couple of quality examples to give you a taste!
Forest
Forest has a simple agenda: to make the dashboard look good. It can control menu items, widgets and the login page, all while looking fabulous with a custom design.
This theme is geared towards eCommerce, and you will find that the menu and widgets have been modified to suit. The settings area has options for changing the appearance of the dashboard as well as the login page. You can also add your custom CSS.
Price: $14 | More Information
Easy Blogging
Forest might want to look fabulous, but Easy Blogging wants to simplify things as far as it can. The theme is especially designed to simplify the Dashboard, which is exactly what we want. As with others, this theme will grant control over menu options, widgets, login page and the dashboard.
Easy Blogging is geared at novice users who want to get their work done, plain and simple, without having to deal with a single shred of complexity. It strips the dashboard down to the essentials of pages, posts and comments, thus making it an area free of possible trouble. If you have a mind to go back to the complexities of the conventional dashboard (especially for admin purposes), you can quit ‘simple mode’ and get to work. It pretty much makes the dashboard foolproof – at least where the possibility of keeping the site safe from accidental damage is concerned.
Price: $19 monthly | More Information
Custom Code the Backend
Let’s finish off with an ambitious suggestion: Create your very own custom theme for the Dashboard (via a plugin). Doing so will give you the greatest possible control over your work and enable you to create a truly safe WordPress backend that (1) you want, and (2) your clients deserve.
The possibilities with such an option are huge. For example, you could create a seamless transition from the WordPress front end to back end, making it less intimidating and much simpler for the client.
I’ll leave it there as a tantalizing suggestion. If you’re interesting in us exploring this topic further, let me know in the comments section below!
Conclusion
The default WordPress back end can be overwhelming for clients – especially novices. Simple mistakes or changes from the client can create big trouble for the website. As such, it’s worth the time and effort – for the client as well as the developer – to delve into methods that make the WordPress back end client-proof. You can great a best-of-both-worlds scenario: The complexity of the dashboard can be hidden away from the client without compromising the power of WordPress.
We have listed several tips for the backend safer for clients in this post, but there are of course many other options. Customized dashboard themes are especially promising – they add more power while hiding the complexity. The dashboard could become a powerhouse with custom widgets and increased functionality for clients.
What measures do you take to simplify the WordPress dashboard and make websites ‘client-proof’? Share with us in the comments section below!
Image courtesy of Voin_Sveta / shutterstock
Hi! Very nice topic! It’s simply very well written and useful!
I was interested on how to create my very own custom theme for the Dashboard. Could you please explain more about that?
Thanks!
🙂
Thank you Tom that share most important tip to improve WordPress security for client. I will add them in my checklist to make sure my plugin or backend code is strong.
Actually,security is very important for our wp site.we should backup our client site regularly also we should check their site security loophole.i got very useful information form this post.thanks for your hard work.
Maybe this is the wrong place for this comment, but how do you go about keeping your layout safe FROM clients?
Especially when using things like Divi and other Visual Composer type layout builders?
How do you keep your client from severely breaking the layout of the site while letting them manage their own content?
Kevin, I don’t think it matters what you do, some clients will change your carefully crafted work into something that looks like a 6 year old did it. They don’t understand that whilst their website may be about them, it’s not for them. It doesn’t matter to them that what you did falls into line with web design practices, and meets the ‘above the fold’ requirements, they want to put their own stamp on it. And let’s face it, some people don’t have very good taste. It drives me crazy.
I agree. You spend a lot of time putting together a nice site. Then the client want’s the ability to add pages and blog posts, etc. Unfortunately, they will never conform to our layout style because they don’t see the site and design like we do. This is the downfall of WP. Even if you give them and Editor Role, they still have access to ruin the site layout and add a bunch of junk. I think WP should add custom options within the role status core. The White Label CMS has options, but if you enable adding pages, there’s really nothing you can do. Even if you train your client. Remember they are paying us to build a site. If they could do it, they wouldn’t be hiring us. Then you want to backlink their site to yours to show off your work. A new client visits the site and thinks this is nice until they start navigating. Only to find out it looks like the client let their 10 year old nephew who likes to draw and play on the ipad get in their and start “playing”. Very frustrating indeed.
After each build, I do a video walkthrough of the site the way I designed it – that way I can show my work when I handed it off. The live site can confirm the layout I did is still in use, but the video shows how it was when it was first created. Makes a nice internal portfolio too: users don’t have to leave your site to see your work.
Thank you for this post!
do you have more explanation for:
1. changing the login page from the default wp-login.php, and
2. adding brute force login protection.
do you use a plugin?
Jimmy,
Brute force is available with most security plugins, including Wordfence, iThemes Security, & JetPack. Read more about JetPack’s offering on WPTavern http://wptavern.com/jetpack-3-4-adds-protection-against-brute-force-attacks
You can use iThemes Security plugin to also change the default wp-login.php file. Just backup your site before messing around with any of the security plugins as the can cause issues depending on your setup.
Remember that hiding the login by changing your URL is a version of security by obscurity, a minimal defense tactic, & many (if not most) attackers will find that page anyway. I still think it’s worth doing, but don’t get a false sense of security from it. Change your salt keys, use strong login credentials, keep regular backups, & follow WP’s codex for additional security hardening tips: http://codex.wordpress.org/Hardening_WordPress
I’d like more tips on these two things, as well!
Perfect timing, as usual, for this article. The WP admin is overwhelming for many of my non-technical clients and I have been wanting to do some research on simplifying it. Thanks for coming to the rescue yet again, ET!
Awesome post! I am in the market for just a thing. I would love to hear more form you guys on the subject if you dig any deeper.
This is a great article. I highly recommend another plugin be added to the list.
AAM- advanced access manager
I use it on all of my sites. You can restrict which menu functions, plugins, pages, posts, widgets, etc. each specific user, or user role has access to. It is a must have for clients. I use AAM along with White Label CMS for a perfect white label experience.
Thanks for the tips. I do like the idea of simplifying the display and disabling certain functions. However, I’m reluctant to white-label or visually change the back end for a couple of reasons. One, it seems more beneficial that clients know what CMS they’re using and the company behind it. Two, if you change the dashboard too much, WordPress training videos and tutorials (such as those from WP101) might be confusing. Have you experienced any downsides?
Just wandering if the coding and plugins work well with Divi. I haven’t used a Divi myself, but I know that ET has a custom dashboard
Yes it works. The ET custom dashboard is just theme options that work great with WordPress ecosystem.